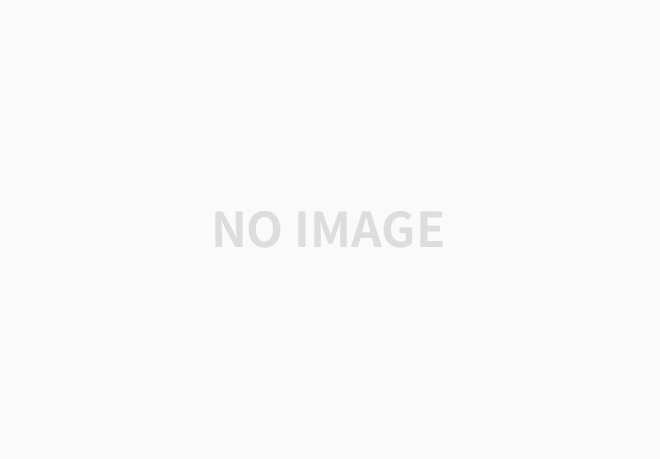
1. 비디오 Collection 만들기
비디오를 올리려면 데이터베이스에 저장을 해야되는데, 아직 그 안에 있는 Tables을 만들어주지 않았다(MongoDB에서는 Collections라고 하는듯)
mySQL로 했을 때는 tables라고 불렀는데 MongoDB에서 부르는 명칭이 살짝살짝 다르다고 한다
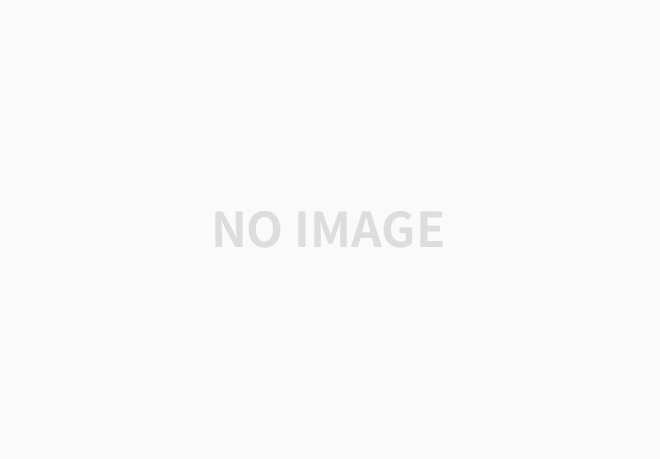
server폴더 - models폴더 안에 Video.js 파일을 새롭게 생성한다
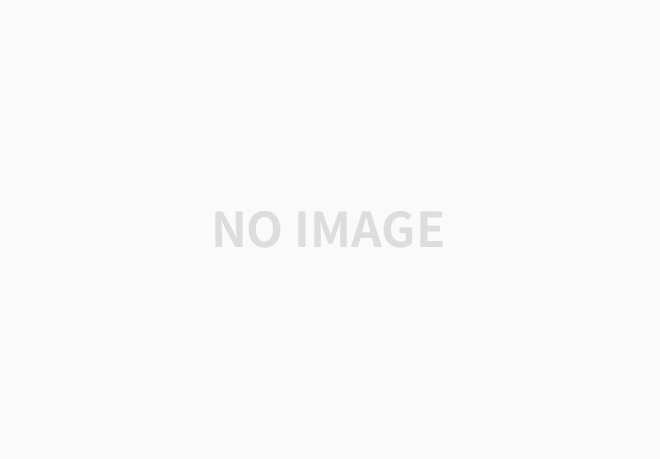
위의 diagram을 보면 writer, title, description, privacy, filePath, category, views, duration, thumbnail을 변수로 넣는다고 했으므로 똑같이 해준다
const Schema = mongoose.Schema;
const mongoose = require('mongoose');
const videoSchema = mongoose.Schema({
writer : {
// 비디오 업로느하는 사람
type: Schema.Types.ObjectId, // writer의 id를 넣음
// schema 형식의 id로 넣으면 User에 있는 모든 정보를 가져올 수 있음(name, email, password ...)
ref: 'User'
},
title : {
tyepe: String,
maxlength: 50
},
description : {
type: String
},
privacy : {
type: Number
},
filePath : {
type: String
},
category : {
type: String
},
views: {
type: Number,
default : 0 // 조회수는 0부터 시작
},
duration : {
type: String
},
thumbnail : {
type: String
}
}, { timestamps : true}) // 만든 날 표시
const Video = mongoose.model('Video', videoSchema);
module.exports = { Video }
************오류 발견************
title에서 maxLength를 설정해줬더니 안된다.. 기능이 없어진 것 같아서 지워줬더니 잘 작동되었다
2. onSubmit 함수 만들기, 3. 요청할 데이터를 서버로 보내기
등록 버튼을 누르면 database에 저장이 되게 하는 onSubmit 함수를 만들 것이다
일단 버튼 눌렀을 때 onSubmit함수가 실행되어야하므로
VideoUploadPage.js에서
<Form onSubmit={onSubmit}>
이러케 해줌
그 전에! 정보들의 이름 확인하자
비디오 업로드 창에서 Redux Devtools를 눌러보면 이렇게 userData가 나온다
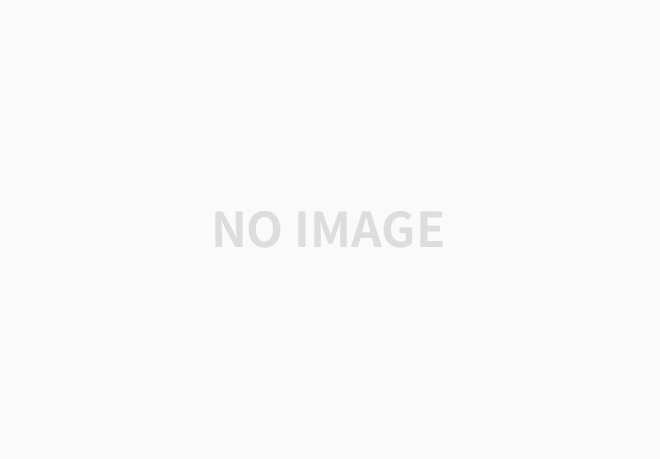
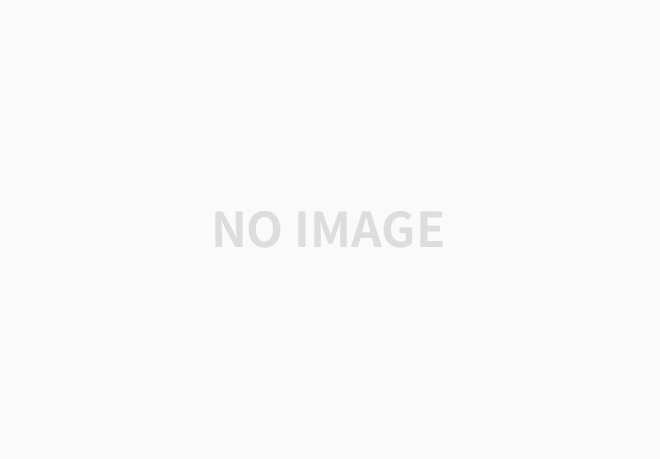
회원가입했을 때 정보들이 저장된 것이다
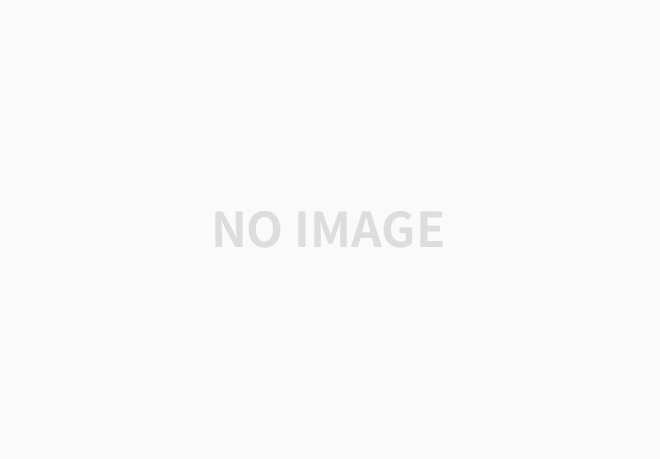
저것들 중에 우리가 넘겨줘야할 정보만 빼서 변수를 만들어 줄 것이다
writer에 id를 넣을건데, 아까 보여준 redux를 이용하여 가져올 것이다
그래서 새롭게 쓸 useSelector를 위해 import를 해줌
import {useSelector} from 'react-redux';
그리고 const user를 생성해주었다 ㅎ
const user = useSelector(state => state.user); // state에 있는 user의 모든 정보를 가져옴
그 다음 onSubmit 함수를 작성한다
const onSubmit = (e) => {
e.preventDefault(); // 우리가 하고 시픈 event를 하기 위해
let variables = { // id를 redux를 통해 가져옴
writer : user.userData._id,
title: VideoTitle,
description: Description,
privacy: Private,
filePath: FilePath,
category : Category,
duration : Duration,
thumbnail: ThumbnailPath
}
Axios.post('/api/video/uploadVideo', variables)
.then(response => {
if(response.data.success){
console.log(response.data)
}
else{
alert('비디오 업로드에 실패했습니다.')
}
})
}
writer를 제외한 것들은 모두 useState로 가져왔기 때문에 저렇게 작성하였다
4. 보낸 데이터를 mongoDB에 저장하기
uploadVideo로 서버에 보냈으니 video.js에 router.post를 써줘야지!
router.post('/uploadVideo', (req, res) => {
// 비디오 정보들 저장
const video = new Video(req.body) // 모든 정보를 담음 . 클라이언트에서 받은 모든 variables가 req.body에 담김
video.save((err, doc) => {
if(err) return res.json({success: false, err})
res.status(200).json({success:true})
}) // mongoDB에 저장
})
아! 저 Video를 쓰기 위해 Video.js를 가져와야 한다
참고로 Video.js에서는 Schema로
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const videoSchema = mongoose.Schema({
writer : {
// 비디오 업로느하는 사람
type: Schema.Types.ObjectId, // writer의 id를 넣음
// schema 형식의 id로 넣으면 User에 있는 모든 정보를 가져올 수 있음(name, email, password ...)
ref: 'User'
},
title : {
tyepe: String,
},
description : {
type: String
},
privacy : {
type: Number
},
filePath : {
type: String
},
category : {
type: String
},
views: {
type: Number,
default : 0 // 조회수는 0부터 시작
},
duration : {
type: String
},
thumbnail : {
type: String
}
}, { timestamps : true}) // 만든 날 표시
const Video = mongoose.model('Video', videoSchema);
module.exports = { Video }
이렇게 작성되어있었다 ㅎ
이거 전체를 가져오기 위해 video.js에서
const { Video } = require("../models/Video");
이렇게 해줬당
그러면
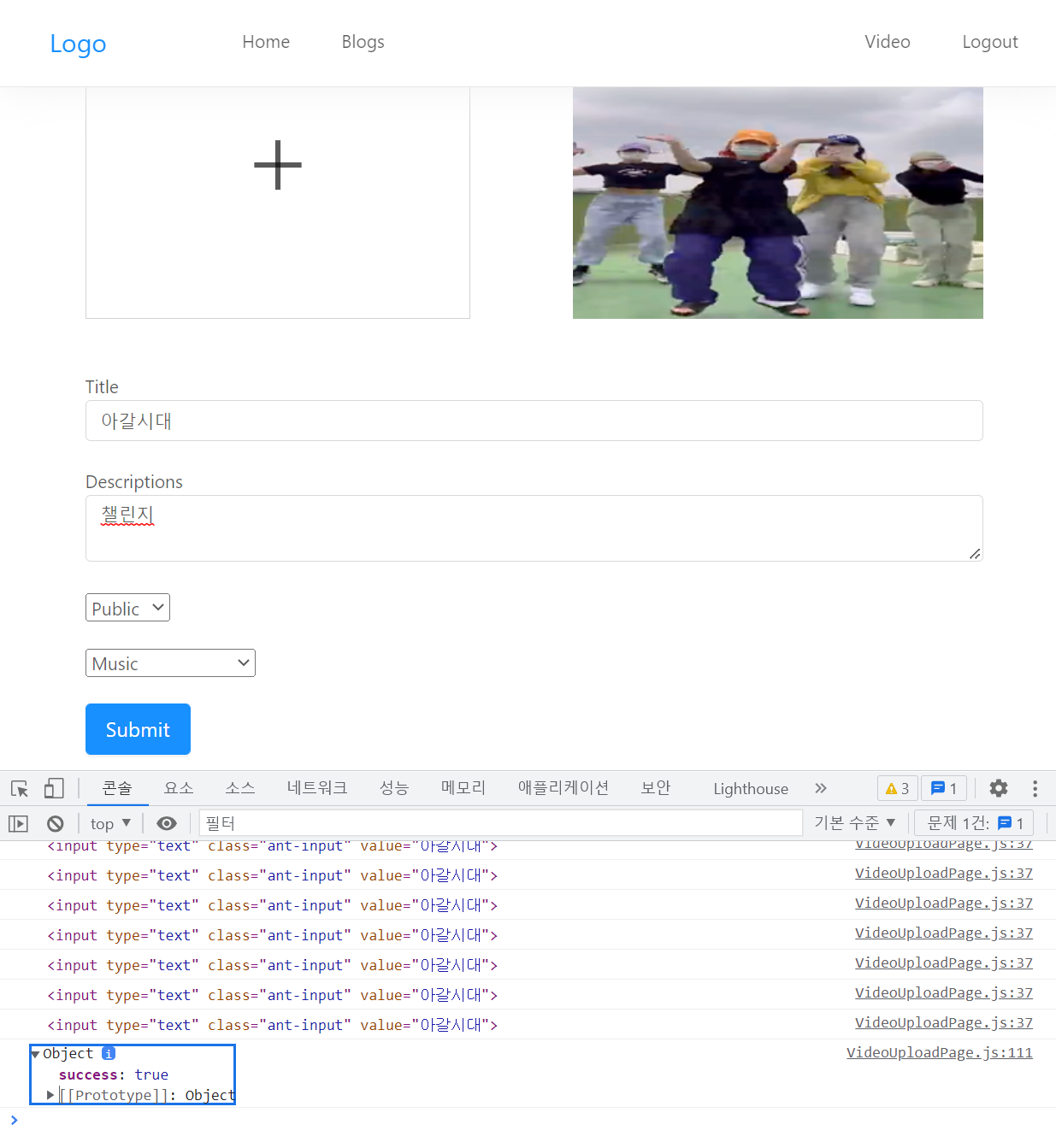
성공이 된 것을 볼 수 있다
아까 video.js에서 save에 성공했을 때 {success:true}만 반환하기로 했으므로 저것만 뜬 것!
우리는 업로드에 성공하면 메세지가 뜨고 난 후 3초 뒤에 landingPage로 돌아가게 만들 것이다
아까 VideoUploadPage.js 에서 onSubmit함수에 코드를 추가한다
Axios.post('/api/video/uploadVideo', variables)
.then(response => {
if(response.data.success){
console.log(response.data)
message.success('성공적으로 업로드 하였습니다.')
setTimeout(() => {
props.history.push('/') // landingpage로 이동
}, 3000)
}
else{
alert('비디오 업로드에 실패했습니다.')
}
})
/만 치면 landingPage로 돌아오기 때문에 저러케 된 것
이러면!
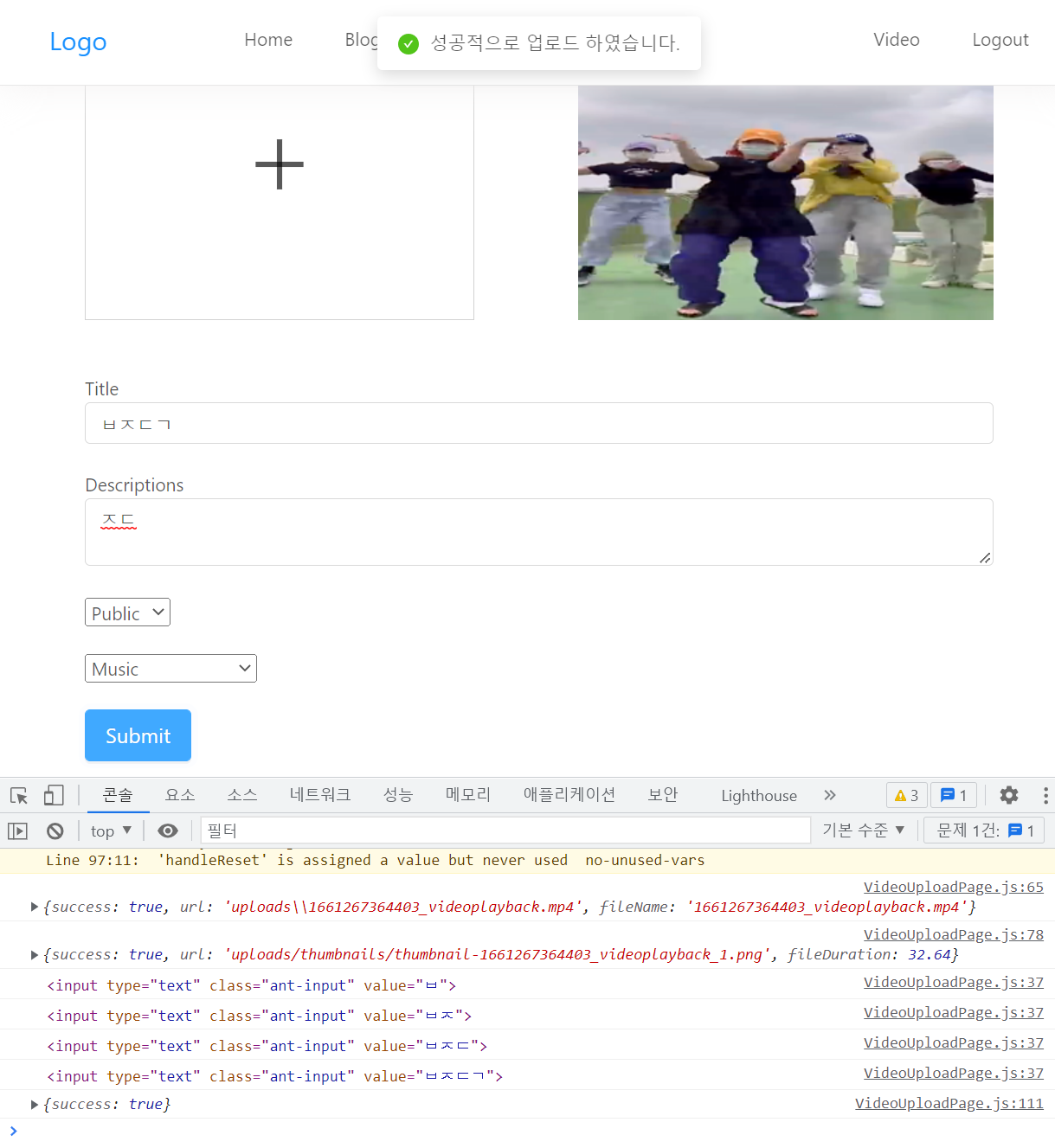
--
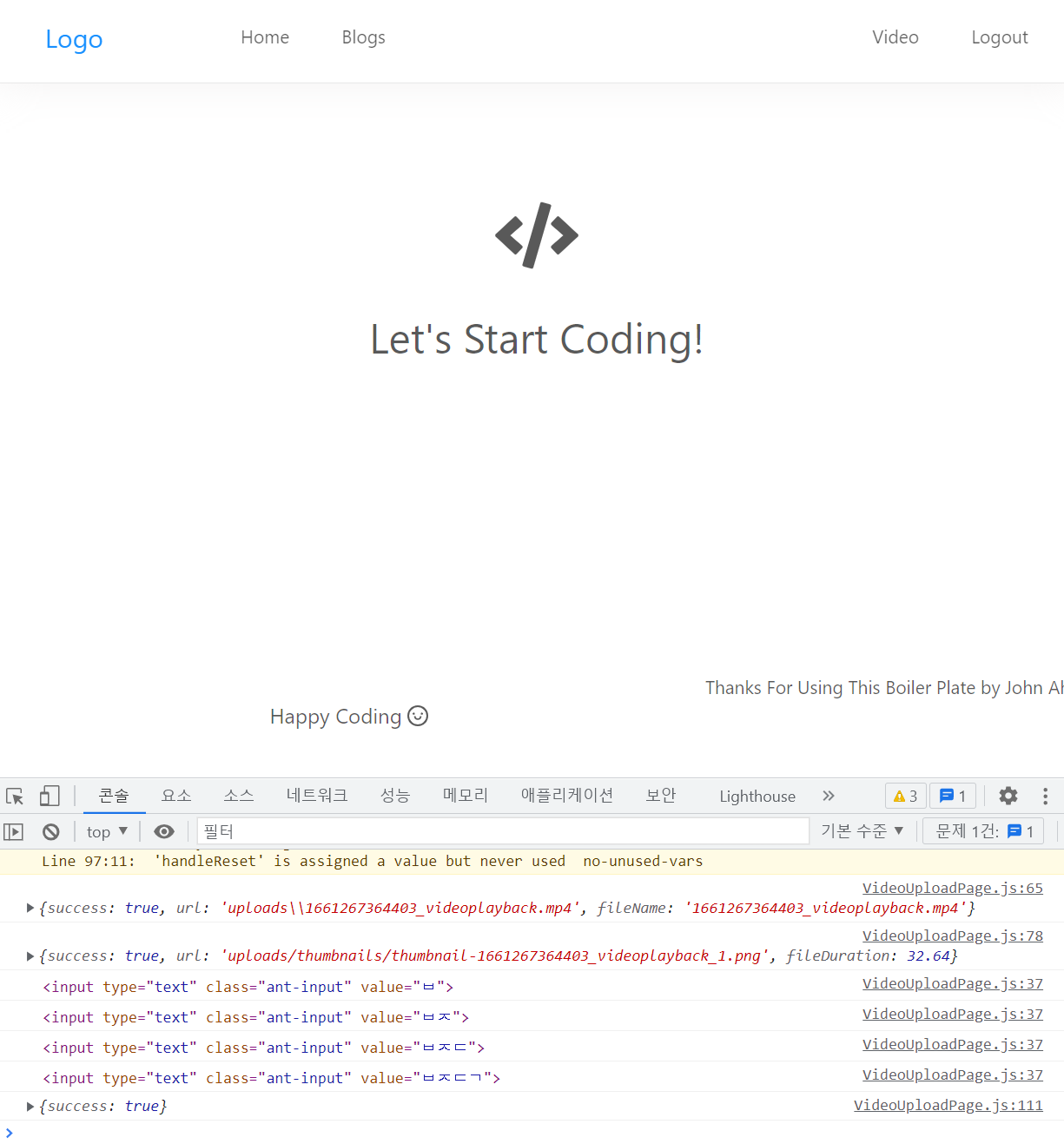
잘 된다 휴우..
mongoDB에서 내가 올린 영상을 확인할 수 있다
Browse Collections로 가서 videos 누르면 됨!
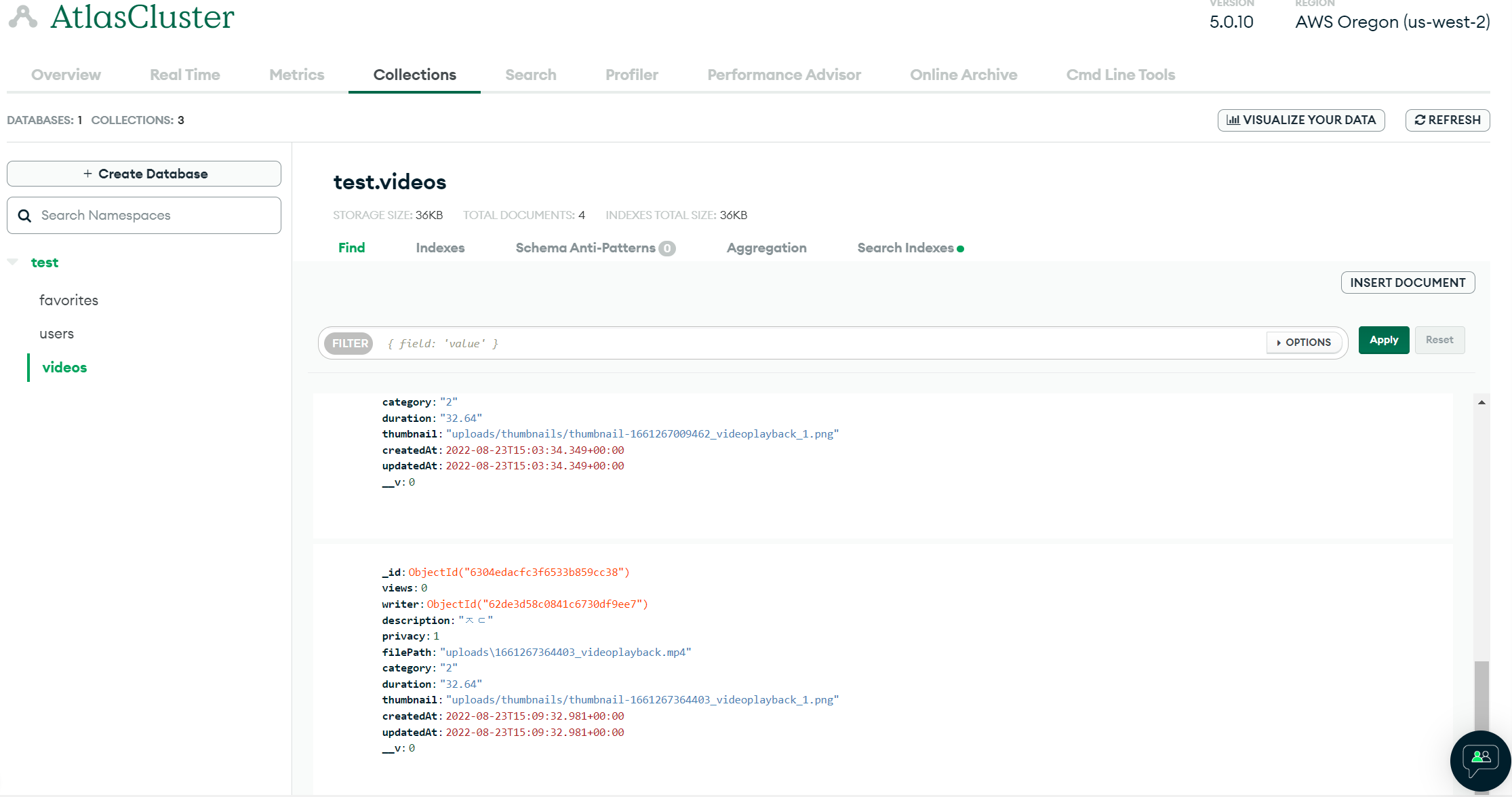
잘 있네요
댓글